Introduction to Nuxt.js and Vue.js
Nuxt.js and Vue.js have emerged as two of the most popular open-source JavaScript frameworks for building modern web applications.
While Vue.js aims to simplify UI development using components, Nuxt.js builds on it further optimizing for challenges like SEO, server-side rendering etc.
In this comprehensive guide, we’ll analyze their features, strengths and weaknesses, appropriate use cases and more across numerous criteria to provide clarity on when to choose one over the other.
By the end, you’ll have sufficient context for making the right framework decision aligned to your needs for assembling your next web project. So let’s get started!
What is Nuxt.js?
Nuxt.js is an open-source framework making web development simple and powerful. Built on top of Vue.js, it eases app development by providing features for common real-world concerns like SEO-friendly pre-rendering, abstraction of complex server configurations, simplified authentication etc. right out of the box through a set of conventions.
It aims to offer an intuitive structure keeping your code maintainable as your app grows while eliminating tedious configuration needs. The goal of Nuxt.js is to allow you to focus on creating amazing user experiences.
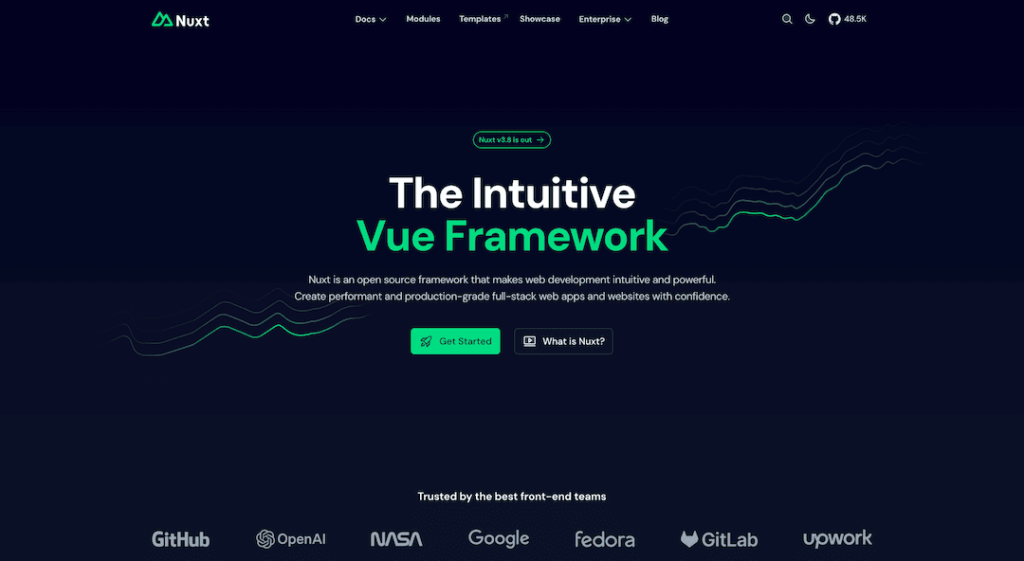
Top 10 Features of Nuxt.js
Here are the most significant capabilities offered by Nuxt.js:
1. Server Side Rendering
Nuxt.js provides built-in SSR support pre-rendering content helping indexability and performance.
2. File-based Routing
Routes are automatically inferred from your app’s filesystem structure eliminating manual routing configuration.
3. Vuex Integration
Nuxt offers direct integration with Vuex state management with auto-registered modules right out of the box.
4. Static Site Generation
In addition to SSR, full static pre-rendering during build for blazing speeds and scalability is also supported.
5. Seamless Authentication
Modules like Auth simplify adding authentication across private pages and API endpoints with little effort.
6. Powerful CLI
Using create-nuxt-app allows quick scaffolding apps customized to needs with ES6, TypeScript, UI frameworks etc.
7. Development Ease
Hot reloading during development and features like accessible process flags provide excellent DX.
8. Module Ecosystem
Nuxt modules allow painless integration with third-party libraries and extended capabilities as needed.
9. Large Community
An active growing community around Nuxt writing tutorials, plugins etc. provides ready help during development.
10. Progressive Web Apps
Nuxt supports building web apps using technologies like service workers or IndexDB that load instantly just like native mobile apps.
5 Use Cases of Nuxt.js
Some popular scenarios where Nuxt excels include:
- Marketing Sites – Nuxt helps in building fast, SEO-friendly marketing websites to reach wider audiences.
- Ecommerce Portals – For high-traffic online stores focused on user experience, Nuxt is fantastic.
- Web Applications – Nuxt eases building feature-rich web apps with great UI/UX leveraging SSR speed.
- Company Homepages – Static sites showing basic company info benefits through Nuxt’s SSG and SPA mode.
- Documentation Platforms – Docs sites render fast leveraging Prerendering search engine bots required.
Top 5 Projects Using Nuxt.js
Here are some well-known projects using Nuxt.js:
- Vue – The official documentation site for Vue at vuejs.org uses Nuxt for its web app.
- GitLab – Some UI aspects of the popular GitLab repositories manager use Nuxt.
- Le Monde – The French newspaper Le Monde utilizes Nuxt to optimize its online news site.
- Sygic Travel – Nuxt powers the Sygic Travel trip planning and bookings web platform.
- DICE – The popular online ticketing site DICE leverages Nuxt to display events.
Performance of Nuxt.js
Nuxt.js incorporates various optimizations for delivering amazing speeds right out of the box:
Server Side Rendering
Pre-rendering content on the server avoids the initial rendering workload for clients allowing quick first paint.
Route-based Code Splitting
Nuxt splits code over routes sending precisely needed chunks and improving time-to-interactive metrics.
Minification & Compression
JavaScript, CSS and HTML automatically compressed bringing down transfer and parse times.
Caching Support
Page or component-specific caching directives intelligently leverage browser caching capabilities avoiding expensive regeneration.
Image Optimization
Integrated image handling ensures responsive sizes, and next-gen formats like WebP are done automatically enhancing perceived load speeds.
So Nuxt incorporates the latest best practices into its architecture choices leading to excellent performance KPIs by default without extra work.
Tooling of Nuxt.js
Nuxt.js offers robust tooling for rapid development:
create-nuxt-app CLI
Quickly scaffold new projects via starter templates customized to include UI frameworks, TypeScript etc eliminating mundane setup.
Hot Module Replacement
See code changes reflect live without needing full refreshes through inbuilt HMR support speeding up iteration.
Vue DevTools Integration
Allowing inspection of Nuxt component trees, routes, state etc through seamless integration with powerful Vue DevTools.
Module Ecosystem Access
Discover and integrate from specialized modules providing cached API requests, markdown rendering etc further boosting DX.
Built-in ES6/ES7 Compilation
Write modern standards-compliant code leaning on integrated Babel pipeline handling compilation of JavaScript down to ES5 during builds.
Example of Nuxt.js
Let’s see a simple Nuxt page outline showing core concepts:
// nuxt.config.js
export default {
modules: ['@nuxtjs/axios'],
css: ['~/assets/css/main.css']
}
// layouts/default.vue
<template>
<Header/>
<Nuxt />
<Footer />
</template>
// pages/about.vue
<template>
<h1>About Us</h1>
</template>
<script>
export default {
async asyncData() {
// Fetch data
}
}
</script>
// store/index.js
export const state = () => ({
count: 0
})
We see file-based routing, programmatic data fetching, layouts, self-contained components compose together into full Nuxt apps.
State Management in Nuxt.js
Nuxt.js offers direct integration with Vuex leveraging auto-registered modules right out of the box for managing state.
The store directory contains Vuex modules split by domain/feature like auth, shopping cart etc avoiding a messy unified blob. this.$store
instance allows simple usage across components.
For smaller apps, built-in useState
may suffice vs the structure Vuex encourages. The choice depends on app complexity – integrating state libraries like Pinia, Vuex avoids prop chains growing unmanageable at scale.
The Pros of Nuxt.js:
Let’s analyze some key strengths powering Nuxt adoption:
- Easy Setup – With CLI scaffolding and sane defaults, your Nuxt app hits the ground running avoiding complex tooling configs.
- Built-in SSR – No need to spend time manually adding and debugging server-side rendering to boost SEO and speed. Nuxt handles it all for you.
- Automatic Code Splitting – Nuxt automatically optimizes performance through code splitting avoiding massive JavaScript bundles.
- Simplified Routing – File-based conventions eliminate need for manually defining routes in Nuxt apps.
- Module Ecosystem – Quickly add capabilities like auth, content management etc. leveraging specialized Nuxt modules.
- Vibrant Community – Numerous tutorials, articles and plugins exist answering common needs speeding up learning.
The Cons of Nuxt.js:
However no framework is without certain tradeoffs for its strengths which are worth acknowledging regarding Nuxt:
- Learning Curve – Understanding concepts like middleware, and programmatic routing needs initial time investment beyond basic Vue usage.
- Least Flexible – Enforcing opinions through conventions causes loss of flexibility available through manual configurations.
- Complex Tooling – Dealing with Node servers, Nginx configs makes productionization beyond prototyping demanding specialized expertise.
- Version Upgrades – Given speed of Nuxt evolution, changes between versions during upgrades causes integration difficulties needing effort.
- Not Mobile Native – No ability to reuse Nuxt code directly natively on mobile platforms unlike React Native for Next.
What is Vue.js?
Vue.js is a hugely popular open source JavaScript framework for crafting modern web application user interfaces. It makes building reactive UI components encapsulating their own state easy leading to maintainable code.
Vue emphasizes a declarative approach through HTML-based templates helping web designers ease into building JavaScript-powered UIs. Simple binding also allows rapidly building MVPs leveraging powerful scaling options as app grows.
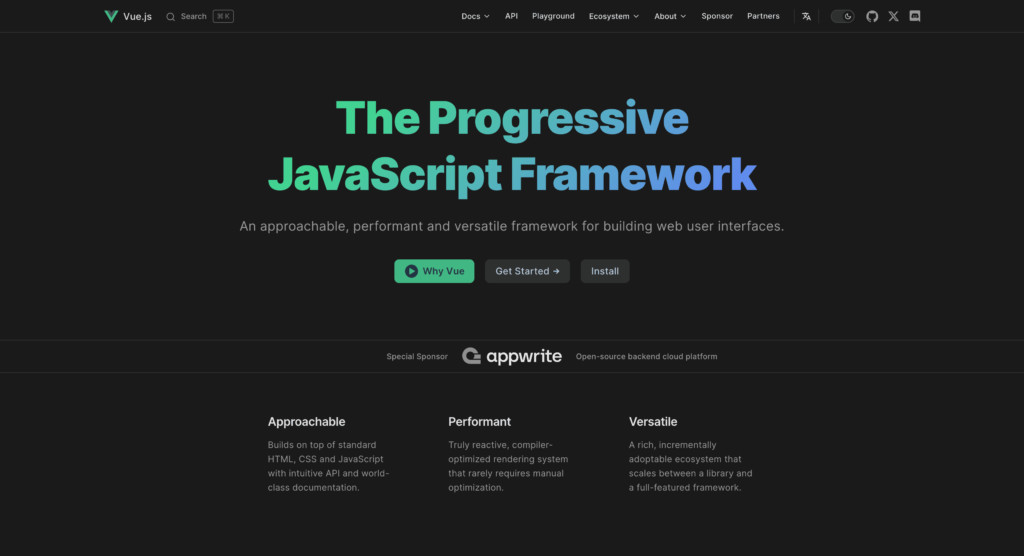
Top 10 Features of Vue.js
Here are the main capabilities fueling Vue’s exponential growth:
1. Virtual DOM
Vue leverages a lightning fast virtual DOM diffing algorithm enabling high performant component updates.
2. Templating via JSX
JSX-like templates allow cleanly expressing a component’s UI and data binding declaratively using familiar HTML.
3. Reactive Components
Vue components reactively track dependencies and update elements intelligently minimizing DOM manipulation.
4. State Management with Vuex
Vuex provides a centralized store helping easily mutate application state predictably.
5. Client-side Routing
The officially supported vue-router offers a routing system for single-page application modes in Vue apps.
6. Component-Based
Building via self-contained component compositions enhances reusability, and testing and maintains separation of concerns.
7. Huge Ecosystem
Robust surrounding ecosystem offers routing, state management solutions complementing Vue’s focus on the view layer.
8. Approachable for Beginners
Intuitive template syntax and a gentle learning curve helps onboarding making Vue very approachable.
9. Lightweight
Vue is very lightweight allowing fast initial loading times coupled with above-average rendering speeds.
10. Great Documentation
Comprehensive documentation and guides make learning Vue structured answering common how-tos for rapid starts.
5 Use Cases of Vue.js
Here are the most popular scenarios where Vue.js delivers superbly:
- Dashboards – For crafting intricate data dashboards with dynamic charts, Vue is great.
- Web Applications – Vue’s flexible component model suits most common web app UI needs.
- Mobile Web Apps – Leveraging capabilities like routing, Vue works smoothly across mobile browsers.
- Small Interactive Elements – Dropdown menus, hover popups etc can be built simply via Vue.
- Rapid Prototyping – Vue’s capability to quickly translate ideas into intuitive interfaces makes iterative prototyping fast.
Top 5 Projects Using Vue.js
Some popular applications using Vue.js include:
- Alibaba – Ecommerce giant Alibaba uses Vue.js extensively in its web apps like Xianyu.
- GitLab – Parts of the popular GitLab dev collaboration tool utilizes Vue.js.
- Laracasts – The Laravel video tutorial site Laracasts is built using Vue for the UI.
- Nintendo – Gaming firm Nintendo’s affiliate web app 2game uses Vue.js for optimal interactivity.
- 9GAG – Leading entertainment social platform 9GAG built its Android app using Vue.
Performance of Vue
Some ways Vue ensures high UI responsiveness despite its overall simplicity:
Virtual DOM Diffing
Vue manipulates actual DOM minimizing only where needed through smart virtual DOM comparisons leading to optimized updates.
Async Component Handling
Code splitting made simpler while keeping user informed of loading state via async components avoiding massive bundles.
Compiled Templates
Underlying rendering functions precompiled avoiding runtime compile costs of string-based templates improving component initialization speeds.
Buffer Based Batching
Updates strategically coalesced together into a single tick through buffering instead of multiple fine-grained changes enhancing observed lag.
So Vue incorporates considered design choices focused on the rendering layer aimed at maximizing end-user responsiveness forming bedrock for extensions handling more.
Tooling of Vue
Vue offers great fully customizable tooling ecosystem instead of enforcing opinions:
Vue CLI
The @vue/cli handle instant app scaffolding via project-specific configs eliminating mundane setup and letting developers focus on building.
Chrome DevTools Extension
Allows inspecting component trees, vitals and debugging capabilities right within Chrome DevTools put through rigorous testing and reliability focus by core team.
Integrated Solutions
Ecosystem tools like Vue Router for routing, Vuex for state management integrate beautifully vs needing to glue third-party options together owing to open nature of Vue architecture.
Vibrant Plugin Ecosystem
Hundreds of specialized plugins catering for needs like TypeScript integration, testing, linting etc provided by the community exist unlike always needing to reinvent internally.
Example of Vue
Here’s a simple Vue component outlining key aspects:
<!-- ComponentTemplate.vue -->
<template>
<div>
<loader v-if="loading"></loader>
<slot v-else></slot>
</div>
</template>
<script>
import loader from './Loader.vue'
export default {
components: {
loader
},
data() {
return {
loading: false
}
}
}
</script>
We see single file encapsulation via script + template, components composed together, reactive bindings etc enabling building complexity starting from intuitive foundations.
State Management in Vue
For simple apps, built-in API like ref, reactive etc may suffice for managing state without needing external libraries.
But for non-trivial apps needing shared state across larger component trees, dedicated state management libraries like:
Vuex – Centralized store for state using mutations, actions, getters etc serving as official recommended library.
Pinia – Provides a store API without verbosity tracking state, getters automatically unlike Vuex’s explicit needs.
These integrate smoothly keeping data flow transparent and debuggable. The choice depends on app scale – integrating state libraries avoids cascading prop chains taxing maintainability.
The Pros of Vue.js:
Let’s explore the strengths that make Vue an excellent framework:
- Lightweight – Vue has one of the smallest bundle sizes among comparable options keeping its footprint small.
- Simplicity – Vue sports one of the most approachable learning curves appealing to new developers trying out web frameworks.
- Great Documentation – Extensive documentation coupled with easy examples helps learn Vue’s paradigms fast.
- Reactive Interfaces – Vue’s efficient reactivity tracking ensures UIs stay updated to state changes.
- Fast Rendering – Intelligently batching DOM updates makes Vue very performant.
- Ease of Integration – Drop Vue incrementally on existing projects with different JavaScript bits unlike full-frameworks requiring total buy-in immediately.
- Flexibility – Vue scales up smoothly from basic needs to complex enterprise setups allowing great breadth of usage despite simplicity.
The Cons of Vue.js:
However there certain limitations around Vue worth keeping in mind:
- Immature Ecosystem – The surrounding Vue ecosystem still trails React significantly in terms of components, helper libraries etc limiting choice.
- Client-side Only – Unlike Nuxt and Next, Vue alone focuses purely on client-side concerns needing extensions for server side rendering.
- More Decisions – Lack of conventions requires manually evaluating and integrating routing, build chains, state management solutions for each new project.
- Template Verboseness – Logic expressed via templates causes verbosity vs JavaScript heavier solutions claiming superior tooling support.
- Limited Browser Support – Depending on polyfills means features like transition hooks don’t work on all browsers unlike standards-based equivalents.
Comparing Nuxt.js and Vue.js: 10 Key Similarities
Now that we have a strong grasp on Nuxt.js and Vue individually, let’s analyze areas where they demonstrate substantial overlap:
1. Vue-based
Nuxt and Vue share deep similarities in template syntax, API available etc remaining tightly coupled to Vue capabilities.
2. Components Core
UI layout via mix-and-match reusable components forms fundamental building block within both Nuxt and Vue.
3. Reactivity Support
State mutations propagate reactively updating tightly integrated UI elements avoiding unnecessary renders in both frameworks.
4. Programmatic Routing
Dynamic route generation via JavaScript helps create parameterized URLs and intricate client-side routes within Nuxt and Vue.
5. Single File Components
Co-locating template, styles and logic needed for self-sufficient components in single files eases development of isolated components.
6. Development Flexibility
Nuxt and Vue allow freedom to various degrees in templating choice, data manipulation means, architecture decisions accommodating preferences.
7. Rich Data Binding
Varied automatic synchronization strategies between state and DOM like one-way, two-way binding cuts programming verbosity within templates.
8. First-class TypeScript
Static types via optional TypeScript integration ensures superior editor tooling support, great autocompletion and error detection in both Nuxt apps and Vue.
9. Direct Manipulation
Imperative DOM access through refs and events complements declarative data flow enabling escape hatches for direct manipulation.
10. Module Extension
Capabilities are enhanced for specialized needs like state management via mix-and-match modules instead of reinventing everything within the core in both Nuxt and Vue.
Comparing Nuxt.js and Vue.js: 10 Key Differences
However, differences do exist across numerous vectors differentiating Nuxt.js and Vue.js:
1. Core Purpose
Vue directly focuses on reactive UIs while Nuxt looks at app-level concerns like SSR, and routing aside from UI, like full frameworks.
2. Execution Context
Vue limits itself exclusively to client while Nuxt switches between server and client during execution depending on rendering needs bringing in complexity.
3. Learning Demand
Small API surface area makes ramp-up smooth with Vue, unlike Nuxt’s greater learning investments for aspects like async data, middleware etc.
4. File Conventions vs Freedom
Enforced conventions exists regarding folder structure for aspects like store, pages within Nuxt providing a structured approach vs flexibility in Vue.
5. Auto-generated vs Manual Routing
File-system based automatic routing eliminates tedious config needs in Nuxt unlike handwritten definitions in Vue needing precision balancing convenience and control.
6. Build Process Handling
Comprehensive build config spanning optimization, modern mode support, transpilation, etc exist out of the box for Nuxt vs fully customizable build process when using Vue.
7. Error Handling
Additional effort needed for graceful error handling on Nuxt server-side vs simpler client-only error boundaries offered by Vue.
8. Ease of Debugging
Vue Devtools coupled with browser capabilities enables superior inspection and debugging capability vs Nuxt split between server and client-side debugging.
9. Ecosystem Maturity
Vue offers superior extension diversity in terms of complementary tools, helper libraries etc vs Nuxt still expanding its supporting ecosystem.
10. Mobile App Development
Reusable cross-platform code between mobile and web exists in Vue via NativeScript unlike focus purely on web alone in case of Nuxt.
The Teams Behind Nuxt.js and Vue.js
The developers supporting the frameworks too showcase notable divergence:
Vue – Vue was created by ex-Google engineer Evan You in 2014 as a side project being now maintained by him full-time along with an expanding team of core contributors all around the world.
Nuxt – Nuxt.js was created in late 2016 by front-end consultant Sébastien Chopin eventually seeing contribution from 503 collaborators at present on GitHub alone at varying intensities.
So we see both frameworks originate from individual creators’ personal needs eventually thriving through worldwide community contributions rather than corporate backing in contrast to options like React, Angular etc.
Of course the commercial ecosystem around Vue successfully securing VC funding is certainly far ahead vs Nuxt still finding equivalent commercialization success.
Here is a table comparing some key differences between Nuxt.js and Vue.js:
Feature | Nuxt.js | Vue.js |
---|---|---|
Type | Framework | Library |
Purpose | Web app framework | View layer library |
Rendering Process | Server-side rendered pages | Client-side only by default |
Performance | Auto-optimized | Manual effort required |
Routing | Automatic, file-system based | Configurable manual setup |
Build Configuration | Conventions based | Flexible procedural configuration |
Bundle Sizes | Smaller bundles | Can have larger bundles |
SEO | Out-of-box SEO friendly | Needs extra SSR setup |
Learning Curve | Steeper initial learning | Gradual ramp up |
Tooling | Batteries included | Flexible ecosystem extensions |
State Management | Integrates Vuex nicely | Agnostic to choose solutions |
Mobile App Development | No official mobile solution | Options like NativeScript exist |
My Personal Opinion
Throughout this comprehensive Nuxt.js vs Vue.js comparison, we evaluated their capabilities across various vectors. Both are incredible frameworks for building web experiences.
However, if I had to pick one for my next web application project, I would personally go with Nuxt.js over just Vue. As someone with Vue experience, Nuxt.js accelerates my productivity even further through its batteries included approach.
The seamless SSR support, automated performance optimization, simplified routing setup and structured conventions provide an excellent developer experience right away without complex toolchain integration needs. I can simply focus on UI development leveraging my Vue skills.
The reduced bundle sizes also provide a snappier user experience improving perceived performance – something vital for modern web experiences. While Vue offers ultimate customizability and flexibility, the out of the box efficiencies Nuxt.js enables helps me deliver complete applications significantly faster aligning well with my style.
So for me, Nuxt.js effectively complements Vue expanding its capabilities for assembling full web applications vs just the view layer in Vue. Of course developers should assess their existing preferences, needs and constraints before choosing. But I’ve found Nuxt.js accelerate development tremendously thanks to its set of well-considered defaults and auto-optimizations.
Conclusion
Nuxt.js and Vue offers powerful front-end solutions catering to slightly distinct needs.
Vue focuses on crafting reactive UIs with simplicity enabling progressive enhancements via its modular approach. Nuxt builds on that for addressing common real-world concerns through conventions trading some flexibility.
For traditional SPA models with dynamic interfaces, Vue itself proves very capable. Nuxt brings additional advantages where SSR, static generation and finely tuned performance matter right from the start.
Evaluate criteria like app type, growth trajectories, team skills and project timelines. Choose the framework aligning best with your needs – both continue strong momentum proving viable options for assembling modern web experiences.