Introduction to Next.js and React.js
Next.js and React.js have emerged as two of the most popular JavaScript frameworks for building modern web applications.
While both leverage the power of React for creating interactive user interfaces, Next.js aims to improve the overall developer experience and end-user performance.
In this comprehensive guide, we’ll delve into all aspects of Next.js and React.js – their features, strengths and weaknesses, use cases, examples etc. – to help you determine which one better suits your needs.
By the end, you’ll have clarity on what sets these two frameworks apart so you can make an informed decision for your next web project. So let’s dive in!
What is Next.js?
Next.js is an open-source development framework built on top of React enabling React-based web applications with server-side rendering and static site generation support.
It aims to provide the best possible developer experience with features like automatic compilation, bundling, file-based routing, API routes and page pre-rendering.
Overall Next.js offers a streamlined structure keeping performance, SEO and scalability at the core while removing tedious configuration needs.
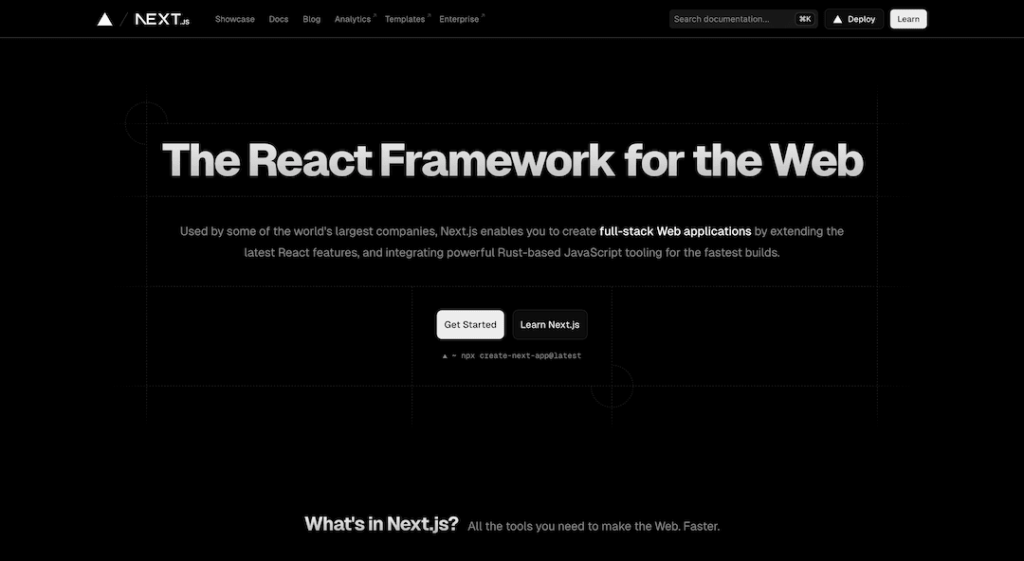
Top 10 Features of Next.js
Here are some of the standout capabilities that make Next.js so popular:
1. Server Side Rendering
Next.js applications come with SSR (Server Side Rendering) enabled out of the box allowing faster initial page loads.
2. Incremental Static Regeneration
Incrementally generate or update static pages, providing the speed of static generation with the dynamic capabilities of SSR.
3. Automatic Code Splitting
Next.js automatically splits code avoiding massive bundles ensuring lean page delivery.
4. Image Optimization
Images are automatically optimized responsive, lazy loaded, WebP converted etc. to boost performance.
5. Fast Refresh
See code changes instantly without losing component state with the Fast Refresh feature enhancing DX.
6. Zero Config Routing
App-based and Page-based routing system removes needs for manual route configuration aligning file system structure.
7. SEO Friendly
Next.js generated pages focus on SEO best practices enhancing optimization for search engine crawling.
8. API Routes
Handle API requests directly within Next.js apps using the integrated API endpoints without complex external server setup.
9. TypeScript Support
First-class TypeScript support options available without needing custom configurations improving type safety.
10. Simplified Production Builds
Complex production build configurations around optimization and more handled automatically by Next.js.
5 Use Cases of Next.js
Here are some of the most popular use cases where Next.js excels:
- Ecommerce Sites – Next.js delivers the performance, SEO capabilities and dynamic support ecommerce sites need.
- Blogs/News Sites – Content focused sites prioritizing speed and discoverability see great benefits from using Next.js.
- Dashboards – Next.js eases building internal tools, analytics panels, admin systems etc. with SSR and APIs.
- Landing Pages – The preprocessing and optimization abilities make Next.js a top choice for landing pages.
- Progressive Web Apps – Next.js enables building web apps with offline support, push notifications etc.
Top 5 Projects Built With Next.js
Notable projects using Next.js include:
- Netflix – Netflix taps Next.js for critical marketing and informational pages to provide great UX.
- Nike – Nike rebuilt its website using Next.js enhancing its ecommerce performance.
- Twitch – The popular game streaming platform relies on Next.js for its scalable web UI.
- Hulu – Video streaming service Hulu uses Next.js to power optimized viewing experiences.
- TikTok – TikTok’s jobs portal uses Next.js for its recruitment marketing site tiktok.com/careers.
Performance of Next.js
Next.js includes extensive performance optimizations and capabilities out of the box to ensure your applications are blazingly fast. Let’s explore some of the major performance benefits:
Automatic Code Splitting
Next.js automatically splits code avoiding massive bundles ensuring only critical code is loaded.
Image Optimization
Images are automatically made responsive, lazy loaded in next-gen formats like WebP and AVIF enhancing performance.
SSR and SSG
Leveraging server side rendering and static generation significantly cuts browser workload and time to first byte.
Prefetching
Next.js prefetches linked pages and data to have it ready in cache improving user navigation experience.
Production Optimization
Built-in performance enhancements like compression, caching, CI analytics provide optimized production code.
Overall Next.js focuses heavily on speed and benchmarks consistently show excellent performance metrics out of the box.
Tooling of Next.js
Next.js offers robust tooling to streamline development and deployments:
Next.js CLI
The create-next-app
CLI tool bootstraps new apps with sensible defaults and options for TypeScript, ESLint etc.
Hot Reloading
Built-in hot module replacement capabilities push changes instantly without needing full refreshes.
Deployment Flexibility
A wide variety of deployment options are available including Vercel, Netlify, AWS and other Node.js based platforms.
Built-in Compiler
No need for manually integrating solutions like Babel and Webpack. Next.js bundles latest standards automatically.
Code Analyzer
Inspect bundle composition on demand and optimize splitting strategies effectively through provided insights.
Rich Ecosystem
There is a thriving ecosystem of plugins and extras to further boost capabilities like SEO, analytics etc.
Example of Next.js
Let’s see a simple Next.js app outline showing core concepts:
// next.config.js
module.exports = {
reactStrictMode: true,
}
// pages/index.js
export default function Home() {
return <h1>My Next.js Homepage</h1>
}
// pages/about.js
export default function About() {
return <h1>About me</h1>
}
// pages/api/user.js
export default function handler(req, res) {
// API logic
res.status(200).json({ name: 'John Doe' })
}
We see usage of the pages directory for routing, API routes, and config handling important initialization and optimizations under the hood.
State Management in Next.js
Next.js leaves state management open without opinions allowing React solutions like Redux Toolkit, Recoil, Jotai etc. Solutions integrate smoothly without interference from SSR mechanisms.
For simplicity, the React Context and useState APIs suffice for state needs in simpler apps. For more predictable global state, external Reactor solutions help manage complexity cleanly as apps grow.
The Pros of Next.js
Let’s explore the key advantages of using Next.js:
- Simplified SSR – Tedious server-side rendering configuration handled automatically by Next.js.
- Faster Performance – Multiple built-in optimizations enhance performance metrics improving UX.
- Easy Routing – File system-based routing eliminates the need for manual route configuration.
- SEO Capabilities – Next.js generated pages focus heavily on SEO optimization best practices.
- Smooth TypeScript Integration – First-class TypeScript incorporation options are available without added configs.
- Rapid Development – Hot reloading, automated builds etc. reduce development downtimes significantly.
- React Focus – Tight integration allows building complex large-scale React-based apps faster.
The Cons of Next.js:
However, there are also some downsides to be aware of about Next.js:
- Version Upgrades – Jumping between major versions can lead to integration and migration issues needing effort.
- Hosting Constraints – Hosting and scaling requires setting up server components unlike fully static SPAs.
- Steeper Learning Curve – Grasping advanced capabilities like SSR and ISR involves initial effort.
- Limited Customization – Following conventions causes loss of some flexibility for greater control.
- Prerendering Challenges – Rendering strategy needs fit regarding content freshness, size etc to maximize benefits.
- JavaScript Reliance – Next.js adds minimal benefit for sites not heavily leveraging dynamic JavaScript.
What is React.js?
React is an incredibly popular open-source JavaScript front-end library for building fast & efficient user interfaces by Facebook and Instagram.
It emphasizes a component-based approach encapsulating functionality into reusable UI elements that manage their own state resulting in dynamic and responsive interfaces.
While React focuses mainly on front-end view building, its rich surrounding ecosystem provides routing, state management and other solutions.
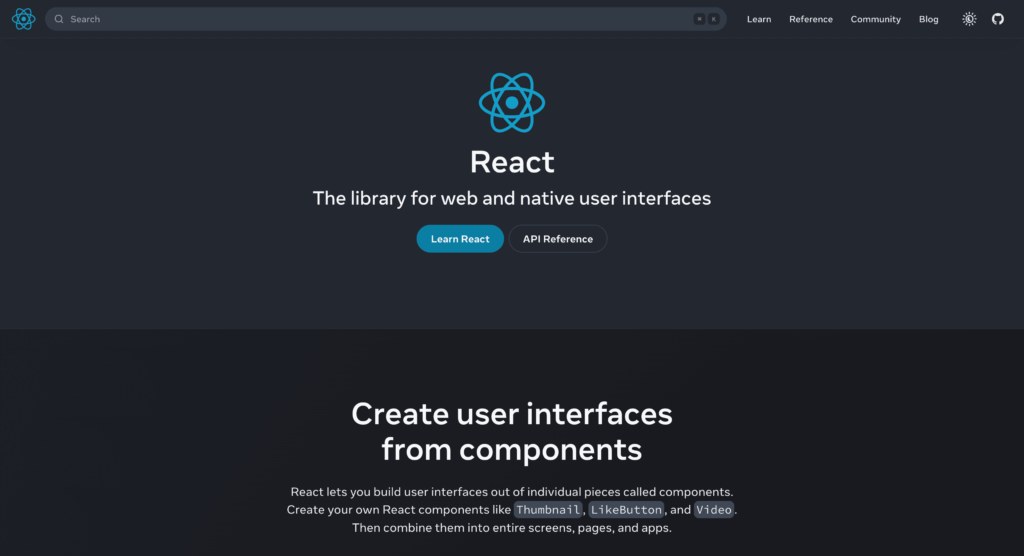
Top 10 Features of React.js
Here are some of the most beneficial features offered by React:
1. Reusable Components
React’s component model allows creating customized reusable UI elements improving reusability.
2. Virtual DOM
The virtual DOM minimizes expensive DOM operations enhancing UI update speeds through intelligent diffs.
3. JSX Support
JSX allows writing markup integrated with JavaScript to define components concisely enhancing developer experience.
4. Uni-Directional Data Flow
Data flows one-way down component trees avoiding tangled flows making state tracking direct.
5. Huge Ecosystem
React benefits from a vast component library ecosystem and surrounding solutions like Redux, React Router etc.
6. React Native
Leverage the same React knowledge to build native iOS and Android mobile apps using React Native.
7. Stateful Components
React Components manage their own localized state using Hooks without complex state management needs.
8. Extensive Community
As one of the most popular libraries, React offers access to rich community resources, tools and help.
9. Stable Codebase
The React code is managed by Facebook and undergoes thorough reliable testing before release into production.
10. Great Documentation
Concise yet comprehensive official documentation covers fundamental concepts to advanced capabilities.
5 Use Cases of React.js
Here are the most popular scenarios where React excels:
- Web Apps – For building intricate web apps with dynamic and interactive UIs, React works great.
- Mobile Apps – Leverage React knowledge to build native mobile apps using React Native.
- Design Systems – Component model suits building an enterprise UI design system.
- Complex UIs – React handles complex UI needs for data dashboards, visualization etc. with a breeze.
- Cross Platform – Write once and reuse across web, mobile, VR using React + React Native.
Top 5 Projects Built With React.js
Notable applications using React include:
- Facebook – The social network uses React extensively in its web and mobile apps.
- Instagram – The popular photo sharing platform is built using React.
- Netflix – Netflix taps React for creating engaging video streaming experiences.
- Dropbox – Cloud storage provider Dropbox rebuilt its web app using React.
- Khan Academy – The popular online education platform Khan Academy uses React for its web app UI.
Performance of React
While React does not automatically optimize performance, some techniques ensure responsiveness:
Virtual DOM
Minimizes expensive DOM updates through intelligent diffing leading to faster UI updates.
Code Splitting
Splitting bundles avoids loading unnecessary code improving payload sizes and page load speeds.
Use Callback
Avoiding inline callbacks leverages reference equality for better memoization reducing pointless re-renders.
React.memo
The React.memo higher order component minimizes re-renders for identical props improving rendering speeds.
So while more manual React allows room for self optimization using above techniques for achieving great UI speeds.
Tooling of React
While React itself remains unopinionated, excellent surrounding tooling ecosystem exists:
create-react-app
The officially supported starter kit includes Babel, ESLint, Jest and Webpack eliminating complex configs.
React Developer Tools
The browser extension provides inspection capabilities to deeply analyze React component structures and diagnose issues.
React Router
The popular community-built solution offers declarative routing avoiding manual URL management.
Next.js CLI
In addition to above, a myriad of libraries for state, styling solutions, UI component kits etc further simplify React development.
Example of React.js
Here is a simple React component showing JSX, state and effects:
import { useState, useEffect } from 'react';
function MyComponent() {
const [data, setData] = useState(null);
useEffect(() => {
fetch('/api/data')
.then(res => res.json())
.then(setData);
}, []);
return (
<div>
<h1>Hello World</h1>
{data && <CustomComponent data={data} />}
</div>
);
}
export default MyComponent;
We see React’s component architecture, hooks for state management and side effects enabling declarative UIs augmented with imperative code.
State Management in React
React offers useState, useReducer for local component state needs. For more extensible global state, dedicated state management libraries like:
Redux Toolkit – Provides easy global store setup, integrates well with React DevTools.
Recoil – Offers shared state atoms closer to React avoiding boilerplate.
Jotai – Primitives for building global state using hooks avoiding Redux complexities.
These integrate smoothly for managing state complexity reducers, selectors avoiding passing state prop drills. Choice ultimately depends on app needs and team preferences.
The Pros of React.js
Let’s explore the major advantages provided by React:
- Simplifies Code Reuse – Components structure promotes reusability avoiding repeated logic across UIs.
- Faster Due To Virtual DOM – Intelligent virtual DOM diffs minimize expensive DOM updates enhancing perceived performance.
- SEO Friendly Rendering – While not default SSR can be added for SEO without full framework change.
- Vibrant Ecosystem – Wide ecosystem of libraries like Redux, React Router provides rich extended functionality with React alone being unopinionated.
- Cross Platform – Write once use across web and mobile using React + React Native avoiding context switching.
- Superior DX – JSX, Flow, and TypeScript improves development experience making building UIs intuitive.
- Stable Code – The React source code itself is thoroughly tested and maintained by skilled Facebook engineers.
The Cons of React.js
However, there are also some downsides associated with React that must be factored in:
- Only Handles Views – React only looks at UI layer needing external solutions for state, routing etc. adding integration complexity.
- Tricky SEO – Out-of-the-box React uses client-side rendering requiring extra SSR setup for SEO optimization.
- Demands Knowledge Investment – The learning curve involves an upfront effort to understand core paradigms like JSX, Virtual DOM, Components etc.
- Versioning Woes – Potential compatibility issues jumping between major versions during upgrades needing careful migration.
- Overchoice Of Solutions – Too many choices for every problem from state to form libraries adds decision fatigue.
- Not Beginner Friendly – The declarative component paradigm shift from earlier paradigms needs an initial grasp adding to onboarding difficulty.
Comparing Next.js and React.js: 10 Key Similarities
Now that we have examined Next.js and React individually, let’s analyze how they line up. We will start by exploring 10 key areas where Next.js and React overlap significantly:
1. Component Focused
Next.js fully leverages the declarative Component paradigm popularized by React.
2. Enhanced DX
Both provide great developer experience through features like hot reloading and minimizing downtime.
3. React Integration
Next.js builds on top of React providing tight integration for easily leveraging React capabilities.
4. TypeScript Support
Optional TypeScript can be added smoothly ensuring type safety without interference for both.
5. Virtual DOM Usage
The virtual DOM diffing provided by React boosts performance for Next.js and React.
6. One Way Data Flow
Uni-directional downward component data flow avoids messy data tangles easing tracking.
7. JSX Syntax Support
JSX syntax for markup integrated with JavaScript code supports defining components concisely.
8. Component Composition
Features are built by combining components enabling large complex UIs by composing simpler components.
9. Vibrant Ecosystem
Both enjoy access to rich surrounding components and helper libraries easing development woes.
10. React Native Compatibility
Leverage shared knowledge to build iOS and Android mobile apps using React Native.
Comparing Next.js and React: 10 Key Differences
However, along with the similarities, there are also notable differences between Next.js and React:
1. Framework vs Library
Next.js provides a full framework while React offers a view layer library needing external solutions.
2. Bundle Size
Next.js applications tend to have lower bundle sizes through code splitting while React requests can get larger without planning.
3. Routing Handling
Routing is defined easily for Next.js apps through the filesystem while React needs a solution like React Router.
4. Build Configuration
Complex production build configs are handled automatically by Next.js vs manually optimizing in React.
5. Server Side Rendering
Next.js offers SSR capabilities enabling pre-rendering where React relies on client side rendering.
6. Static Site Generation
Next.js supports SSG using techniques like ISR allowing partial and full static generation while React applications have client side nature.
7. SEO Strategy
SEO considerations are built into Next.js apps vs additional implementation effort needed with React.
8. Setup Effort
Minimal setup and go vs greater initial configuration and customization demand with React alone.
9. Learning Curve
Next.js offers easier onboarding leaning on React vs grasping declarative React paradigm shift.
10. Updates & Support
Vercel actively maintains Next.js vs Facebook backing React but on longer release cycles.
The Teams Behind Next.js and React.js
The creators supporting the frameworks are also quite distinct:
Next.js – Next.js is actively maintained by Vercel, the web platform company behind the popular Vercel deployment platform created by Guillermo Rauch.
React – React was created at Facebook by Jordan Walke and continues robust development by Facebook, Instagram and open source community contributions.
Next.js benefits from Vercel’s commercial backing and close integration with the Vercel platform. On the other hand, React enjoys Facebook scale adoption, testing and implementation in top apps like Facebook and Instagram.
Both have exceptional community support as reflected by the incredible popularity both enjoy at present.
Here is a table comparing some key differences between Next.js and React.js:
Feature | Next.js | React.js |
---|---|---|
Type | Framework | Library |
Purpose | App Framework | View Layer |
Performance | Auto-optimized | Manual effort needed |
Routing | Built-in, file-system based | Needs external solution |
SSR Support | Built-in SSR | SSR needs setup |
SSG Support | ISR provides hybrid SSG+SSR | Client-side only |
SEO Considerations | Out-of-box SEO-friendly | Extra effort required |
Bundle Sizes | Smaller bundles | Can have larger bundles |
Setup Effort | Zero config setup | Greater config needs |
Style Integration | Built-in CSS support | Needs solutions like CSS-in-JS |
DX | Excellent DX with Fast Refresh etc | React alone provides view layer DX |
Release Cycles | Faster releases from Vercel | Slower more stable releases |
Conclusion
Next.js and React offer incredible front-end solutions for crafting modern web experiences. React focuses on providing an intuitive view layer while Next.js looks to simplify common real world concerns through SSR, routing and optimizations.
For greenfield web apps where speed and discoverability matter, Next.js delivers powerful optimizations enabling great performance right away. React on the other hand suits gradual adoption for improving existing apps allowing adding capabilities over time.
Evaluate your team’s skills, project use case and constraints like timelines to determine what resonates best. Both continue strong momentum so you can be confident selecting either Next.js or React for assembling delightful web experiences.