Introduction to Nuxt.js and Gatsby.js
Nuxt.js and Gatsby.js have emerged as two of the most popular open-source frameworks for building modern web applications. Both leverage powerful web technologies to make development easier, faster and more intuitive.
In this comprehensive guide, we’ll explore all aspects of Nuxt.js and Gatsby.js – their features, strengths and weaknesses, use cases, examples etc – to help you decide which one better suits your needs.
By the end, you’ll have clarity on what sets these two frameworks apart so you can make an informed decision for your next web project. So let’s get started!
What is Nuxt.js?
Nuxt.js is an open-source Vue framework that helps web developers build complex, fast, and universal Vue applications quickly. It is a meta-framework built on top of libraries like Vue, Vue-Router, Vuex etc.
The goal of Nuxt is to make web development feel simpler and more enjoyable with features like automatic coding splitting, file-based routing, hot module replacement and server-side rendering right out of the box.
Overall, Nuxt aims to provide an organized and extendable structure for your codebase while removing tedious configuration needs.
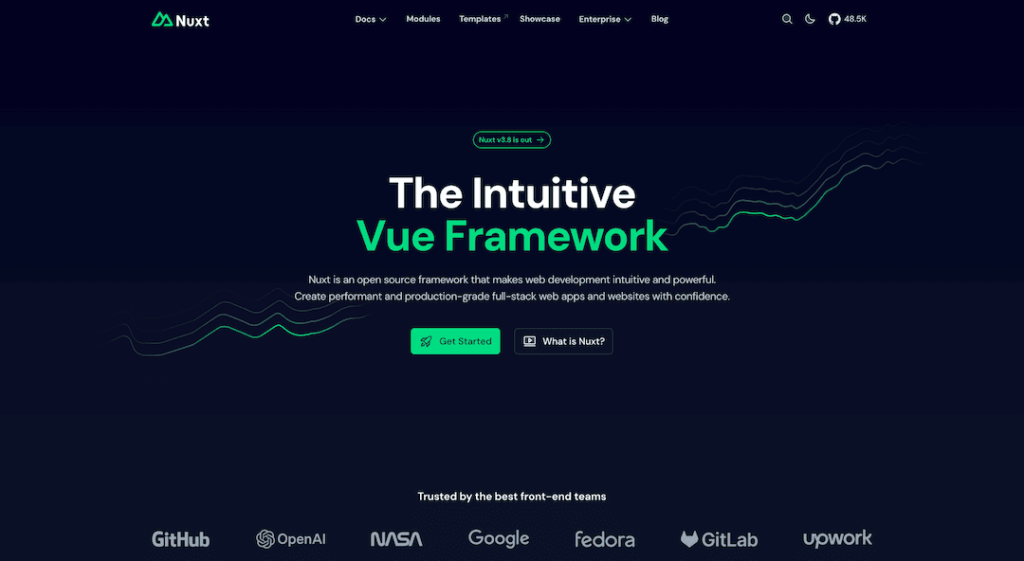
Top 10 Features of Nuxt.js
Here are some of the most useful capabilities provided by Nuxt.js:
1. Vue.js Integration
Nuxt offers deep integration with Vue, allowing building powerful Vue apps and leveraging features like components, directives etc.
2. Server Side Rendering
Nuxt provides built-in SSR support for universal apps that are SEO-friendly. Tedious SSR configuration needs are abstracted away.
3. File-based Routing
Routes are generated automatically based on your file and directory structure removing manual route creation.
4. Vuex Integration
Nuxt offers out-of-the-box integration with Vue’s state management library Vuex for reactive state across components.
5. Code Splitting
Nuxt automatically splits code into separate bundles loaded on-demand instead of one large file improving performance.
6. Hot-reloading Development
Nuxt hot reloads your changes instantly without full refreshes drastically improving dev experience.
7. Static Site Generation
In addition to SSR, Nuxt also supports static site generation for pre-rendered sites offering better speeds and SEO.
8. Module System
Modules extend Nuxt functionality easily integrating tools like Tailwind CSS, Axios etc. without much work.
9. Developer Experience
Nuxt offers an excellent DX with features like automatic imports, aliases etc. accelerating development.
10. Vibrant Ecosystem
There are hundreds of modules built by the Nuxt community available for integrating additional tools.
5 Use Cases of Nuxt.js
Here are some of the most popular scenarios where Nuxt.js excels:
- Marketing Websites – Nuxt.js is great for building fast, SEO-friendly marketing sites.
- Blogs & Portfolios – Nuxt shines for text/image-heavy sites like blogs and portfolios.
- Ecommerce Stores – Nuxt.js simplifies creating high-performance e-commerce platforms.
- Web Applications – Interactive web apps with great UI/UX leverage Nuxt.js capabilities.
- Documentation Sites – Nuxt is a great fit for developer docs sites requiring good DX.
Top 5 Projects Built With Nuxt.js
Some popular projects using Nuxt.js include:
- Vue – The documentation site for Vue at vuejs.org is built using Nuxt.
- Sygic Travel – Sygic Travel’s trip planning & booking site uses Nuxt.
- Le Monde – The popular French newspaper Le Monde relies on Nuxt for its site.
- Hulu – Video streaming giant Hulu uses Nuxt.js to power their web platform.
- GitLab – Parts of GitLab’s UI use Nuxt for optimal performance.
Performance of Nuxt.js
Nuxt includes optimizations for delivering amazing performance right out of the box:
Server Side Rendering
Pre-renders initial shell providing quick first paint even before work done by client.
Route-Based Code Splitting
Nuxt splits code ensuring only what’s needed is sent improving TTI.
Image Handling
Images are automatically made responsive and lazy-loaded for faster loading.
Minification
HTML, CSS and JS are minified in production mode improving payload sizes.
Caching
Assets are cached after initial request removing repeat resource downloads.
Thanks to SSR and other intelligent choices, Nuxt.js consistently benchmarks highly on performance metrics.
Tooling of Nuxt.js
Nuxt.js offers robust tooling for rapid development:
create-nuxt-app CLI
Handles initiating Nuxt projects with specialized configuration needs.
Hot Module Replacement
Make live changes without full refreshes for amazing dev experience through HMR.
Code Analyzer
Inspect bundle composition on demand and fine-tune optimization needs.
Deployment Flexibility
Deploy on any platform supporting Node.js like Vercel, Netlify and more.
Module Ecosystem
A wide array of specialized modules provide added optimizing functionality.
Strong tooling supports makes building and deploying Nuxt apps simple.
Example of Nuxt.js
Here is a simple Nuxt site outline showing usage:
// nuxt.config.js
export default {
modules: ['@nuxtjs/axios'],
buildModules: ['@nuxtjs/tailwindcss']
}
// pages/index.vue
<template>
<h1>Home</h1>
</template>
<script>
export default {
async asyncData({ $axios }) {
const data = await $axios.$get('/api/posts')
return { posts: data }
}
}
</script>
// pages/about.vue
<template>
<h1>About</h1>
</template>
We see Nuxt concepts like asynchronous data fetching, plugins integrated and leaning on Vue SFC structure. Additional optimizations and architectures build on this foundation.
State Management in Nuxt.js
Nuxt.js is tightly integrated with Vuex for state management right out of the box. The store directory registers Vuex modules automatically without boilerplate.
Components can use features like mapState
, mapGetters
etc. for simple access to reactive central state. Actions handle asynchronously manipulating state.
The integration handles universal fetching on server and client seamlessly. Nuxt apps can scale complexity easily as Vuex helps avoid deeply nested component props.
For smaller apps composing state logic declaratively may be simpler without integration. But Nuxt+Vuex together enable straightforward state management at scale.
The Pros of Nuxt.js
Let’s explore the key advantages of using Nuxt.js:
- Simpler SSR – Tedious server-side rendering configs are abstracted away by Nuxt.
- SEO Friendly – Pre-rendering pages help content get indexed easily for search engine crawlers.
- Automatic Routing – File-based routing removes need for manually configuring routes.
- Hot Reloading – Nuxt hot reloads changes in seconds without slowing you down.
- Vue Ecosystem – Tight Vue integration allows building complex Vue apps faster.
- Module Ecosystem – Plug-and-play modules expand functionality easily.
- Organized Code – Enforces a consistent structure keeping code maintainable.
- Vuex Integration – Nuxt offers direct integration with central Vue state management.
The Cons of Nuxt.js
However, there are also some downsides to be aware of about Nuxt:
- Server Setup – Hosting and scaling Nuxt requires additional server configurations.
- Conventions vs Flexibility – Following Nuxt conventions causes loss of some flexibility.
- Interoperability Issues – Integrating non-Vue libraries can take extra effort.
- Version Upgrades – Upgrading between major Nuxt versions can be challenging.
- Template Reliance – Heavy usage of templates over JavaScript comes with a learning curve.
- SEO Rendering Limits – Dynamically updated SEO-unfriendly content hampers effectiveness.
- Not Mobile Native – No official Nuxt mobile solution unlike React Native for Next.
What is Gatsby.js?
Gatsby.js is a free and open-source framework based on React that helps developers build blazing-fast websites and applications. It makes use of modern web technologies like React, GraphQL, and Webpack to create progressive web apps.
The key goal of Gatsby is to enable React developers to build sites that load super fast through pre-rendering pages using static site generation (SSG). All the optimized performance best practices are built-in.
Also, the rich Gatsby plugin ecosystem provides easy integration with other tools like analytics, ads, SEO optimization etc. without much work.
Overall, Gatsby brings the latest web advances together providing an amazing DX tailored for front-end devs.
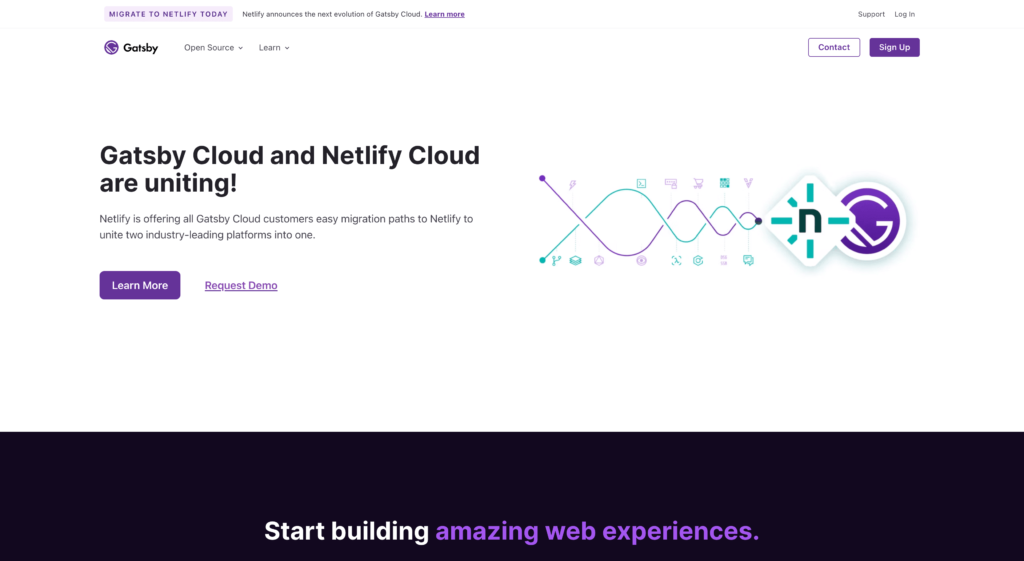
Top 10 Features of Gatsby.js
Let’s take a closer look at the capabilities making Gatsby.js so popular:
1. React.js Focus
Gatsby is purpose-built for using the latest React capabilities giving your sites great interactivity.
2. GraphQL Data Layer
Gatsby uses GraphQL to pull data from different sources into React components on pages.
3. Speed & Performance
Gatsby supercharges performance through built-in optimizations like image processing, lazy loading, code-splitting etc.
4. Plugin Ecosystem
There are thousands of Gatsby plugins for adding functionality like forms, analytics, styling etc. with little effort.
5. PWA Support
Gatsby sites load almost instantly on all devices with features like offline support, caching and manifest generation.
6. SEO Friendliness
Prerendering page HTML ensures content is easily understandable by search engine crawlers.
7. Incremental Builds
Gatsby intelligently rebuilds only changed content and pages minimizing build times significantly.
8. Accessibility Aware
Gatsby incorporates latest web accessibility standards automatically producing inclusive sites.
9. Developer Experience
A great DX is enabled through APIs, CLI tooling, caching, APIs and more accelerating development.
10. Static Site Generation
Gatsby prebuilds a static version of the entire site at deployment time for lighting speeds and scalability.
5 Use Cases of Gatsby.js
Let’s look at the most popular scenarios where Gatsby shines:
- Blogs & Portfolios – Gatsby is fantastic for building text/image-heavy sites like blogs fast.
- Documentation Sites – Speed is vital for dev doc sites which Gatsby provides effectively.
- Company Websites – Gatsby helps companies build blazing corporate sites that impress visitors.
- Marketing Websites – For digital marketing sites, Gatsby delivers the performance that matters.
- News/Magazine Sites – Quick loads keep visitors engaged on news/magazine sites powered by Gatsby.
Top 5 Projects Built With Gatsby
Notable projects using Gatsby include:
- Impossible Foods – To showcase its alternative food products online, Impossible Foods chose Gatsby.
- Nike – Nike’s marketing website is powered by Gatsby for the speed and scalability it provides.
- Figma – Design giant Figma uses Gatsby to ensure its site feels as amazing as its product.
- Gatsby – Gatsby’s own site gatsbyjs.com uses Gatsby and acts as a showcase for it.
- Airbnb Engineering – The Airbnb Engineering Education site uses Gatsby’s capabilities to benefit students.
Performance of Gatsby.js
Gatsby.js builds blazing-fast performance into its core architecture through various optimizations:
Pre-rendering
Gatsby pre-builds the entire site enabling serving static files for speed.
Code Splitting
Code is split ensuring only critical chunks are loaded improving TTI.
Image Optimization
Images are lazy loaded in next-gen formats like WebP automatically enhancing performance.
Caching
All site resources are cached removing expensive data requests after initial load.
Prefetching
Resources for adjacent pages are prefetched enabling instant browsing.
Overall Gatsby benchmarks consistently show incredibly fast site speeds even on complex real-world sites due to its performance focus.
Tooling of Gatsby.js
Gatsby offers excellent tooling for streamlined website building:
CLI Tool
Handles scaffolding sites, running dev environments and production builds etc.
GraphQL IDE
Built-in IDE allows prototyping queries against data right from Gatsby site.
Preview Mode
Make CMS content changes and preview immediately before going live.
Incremental Builds
Only rebuilt pages and assets are recompiled optimizing build speeds.
Plugin Library
Discover and add plugins providing additional functionality easily.
Robust tooling is available covering the whole Gatsby site development workflow.
Example of Gatsby.js
Here’s a simple Gatsby site sample showing key concepts:
// gatsby-config.js
module.exports = {
plugins: [
`gatsby-plugin-image`,
`gatsby-plugin-sharp`
],
}
// src/pages/index.js
import React from "react";
import { StaticImage } from "gatsby-plugin-image";
export default function Home() {
return (
<main>
<StaticImage
alt="Example"
src="../images/example.png"
/>
<h1>Home Page</h1>
</main>
);
}
// src/pages/about.js
export default function About() {
return <h1>About Page</h1>;
}
We see usage of plugins, GraphQL queries, React components all working together in Gatsby. Build tooling and performance optimizations wrap it all together into a blazing fast site.
State Management in Gatsby
For small Gatsby sites built-in React state management like useState
and useContext
may suffice.
But for larger dynamic sites with complex state requirements, integrating state management libraries like Redux is recommended.
The gatsby-plugin-react-redux
plugin smoothly sets up Redux with Gatsby sites handling server rendering, client rehydration etc automatically. Components can dispatch Redux actions and select state values using hooks.
Overall Redux helps manage application state in more complex Gatsby sites avoiding prop drilling and provides centralized stores. The integration ensures it plays nicely with Gatsby’s architecture.
The Pros of Gatsby
Let’s explore the key advantages that make Gatsby so appealing:
- Blazing Speed – The pre-rendered pages result in incredibly fast load times.
- SEO Friendly – Easy-to-crawl structured data helps content rank well on search engines.
- Future Proof – Constant Gatsby upgrades ensure you benefit from the latest browser capabilities.
- Scalable – Hosting Gatsby’s static files is a breeze enabling smooth scaling.
- Vibrant Ecosystem – Diverse functionality is available via the rich plugin ecosystem.
- Advanced Features – Modern web capabilities like offline support, asset optimization etc. built-in.
- GraphQL Agnostic – Fetch data from any GraphQL endpoint not just default source.
The Cons of Gatsby
However, there are also some downsides to using Gatsby that should be factored in:
- Build Delays – Developing and building very large, complex sites can take considerable time.
- GraphQL Learning Curve – Using GraphQL adds initial complexity vs traditional approaches.
- Limited CMS Options – Integration issues exist with some headless CMS tools.
- Multi-Platform Publishing – Publishing across platforms like mobile apps needs custom work.
- Hosting Constraints – Options are more limited compared to hosting regular websites.
- Debugging Challenges – Debugging production issues can pose difficulties with static sites.
- Manual Server Setup – Hosting Gatsby sites yourself requires setting up servers.
Comparing Nuxt.js and Gatsby.js: 10 Key Similarities
Now that we’ve explored Nuxt.js and Gatsby.js individually, let’s analyze how they compare. We’ll start with 10 major points where Nuxt and Gatsby overlap:
1. Open Source Frameworks
Nuxt.js and Gatsby.js are both freely available open-source frameworks with an MIT license.
2. Hybrid Rendering Support
Both support server-side & static site rendering ensuring speed along with dynamic capability.
3. React/Vue Based
While Nuxt uses Vue, and Gatsby uses React, they leverage these popular component-based UI libraries.
4. SEO Friendly
Generating static sites & pages means Nuxt & Gatsby produce SEO-friendly structures crawlers understand.
5. Automated Performance Optimization
They incorporate leading web performance best practices automatically improving speed.
6. GraphQL Integration
GraphQL data layers integrate smoothly empowering pulling data from APIs etc. without much work.
7. Huge Plugin Ecosystem
Having plugins installable with ease enables extending core functionality like forms, auth etc.
8. CLI Scaffolding
Getting started building sites rapidly is easy via CLIs like create-nuxt-app
and gatsby new
.
9. Hot Reloading Capability
Previewing changes instantly speeds up development without needing full refreshes.
10. Easy Deployment
Building & deploying on platforms like Vercel, Netlify etc. is simple allowing hosting sites effortlessly.
Comparing Nuxt.js and Gatsby.js: 10 Key Differences
While Nuxt & Gatsby have their similarities, they also have distinct differences developers should factor in:
1. Framework of Choice
Nuxt is tailored for Vue while Gatsby is focused on React so choice depends on comfort with these.
2. Philosophy
Nuxt prefers convention while Gatsby offers more flexibility needing explicit configuration.
3. Primary Use Case
Nuxt excels at server rendered apps while Gatsby targets lightning fast static sites.
4. Data Sourcing and Transformation Flow
Gatsby uses GraphQL to map and manipulate all data centrally vs more flexibility with Nuxt.
5. Codebase Structure
Nuxt enforces strict organization standards using conventions while structure is customizable in Gatsby.
6. Rendering Engine
Gatsby builds entire project website while Nuxt builds pages as requested so faster incremental rebuilding.
7. Learning Curve
Gatsby’s data layer adds initial complexity vs Nuxt simplifying data flows handled by frameworks likes Vuex.
8. Server Side Rendering
Nuxt has advanced SSR built-in while Gatsby focuses on static site usage over server side generation.
9. Plugin Approaches
Gatsby uses plugins for core functionality vs Nuxt relying on rich frameworks like Vuex for development.
10. App Transportability
Gatsby sites live in the browser so mobile apps need custom integration vs Nuxt is mobile-ready.
The Teams Behind Nuxt.js and Gatsby
The creators driving the frameworks are quite different as well.
Nuxt.js – Nuxt was created in 2016 by front-end developer Sébastien Chopin (@Atinux) and Vue community leader Alexandre Chopin (@alexchopin). They have raised $8 million in funding and continue to push Nuxt’s capabilities forward.
Gatsby – Gatsby was created by Kyle Mathews in 2015. Based in San Francisco, Gatsby raised $55 million in funding before being acquired by Netlify for $700M recently. They offer commercial services & support around Gatsby.
So Nuxt is driven more by an open source collective while Gatsby has strong corporate direction now allowing enterprise direction and growth. The frameworks themselves continue thriving.
Here is a table comparing some key differences between Gatsby.js and Nuxt.js:
Feature | Gatsby.js | Nuxt.js |
---|---|---|
Framework | React | Vue |
Primary Focus | Simpler initial ramp-up | Universal, server-rendered apps |
Rendering Approach | Pre-rendering pages | Server-side rendering |
Data Sourcing | GraphQL data layer | Agnostic data fetching |
Build Process | Entire site pre-built | Pages built as requested |
Performance | Optimized by default | Configuration required |
Plugins | Rich plugin ecosystem | Modules extend functionality |
Learning Curve | GraphQL adds complexity | Simpler initial ramp up |
SEO | Excellent SEO support | Also SEO-friendly |
Folder Structure | Flexible organization | Strict conventions |
Customization | Highly customizable | Can be more constrained |
SSG/SSR | Primary SSG focus | Advanced SSR capabilities |
Mobile Apps | No official mobile solution | Native mobile support |
My Personal Opinion
Throughout this comprehensive comparison between Gatsby.js and Nuxt.js, we analyzed their capabilities, strengths and limitations across a variety of criteria.
If I had to choose one for building my next web application, I would opt for Gatsby.js over Nuxt.js even though I have more familiarity with the React ecosystem.
The main driver is that the blazing-fast performance Gatsby delivers out of the box matters greatly for the types of sites I build. The automatic optimizations and simplified data layer also accelerate my development process.
While Nuxt.js has great merits as well, the concerns around hosting, scaling and needing more configuration for performance are blockers for my use cases where speed is the top priority.
Pre-rendered pages, built-in image handling and seamless PWA features give Gatsby.js sites quite an edge. Its plugin ecosystem also allows extending functionality easily compared to more constrained module approaches.
While both are powerful tools, Gatsby’s performance focus resonates most with my application needs. Of course Nuxt.js can also achieve great speed – but Gatsby.js gives it to me right away benefiting visitors and SEO. So Gatsby gets my vote as the framework of choice.
My Personal Opinion
Throughout this comprehensive Next.js vs React.js comparison, we evaluated their capabilities across a range of criteria – speed, SEO, routing, state management and more.
If I had to choose one for my next web application, I would personally go with Next.js over just React. As someone with React experience, Next.js improves my productivity even further through its extensive batteries-included approach.
The zero config setup, optimized performance, built-in SSR capabilities, simplified routing model and seamless style integration provide an amazing developer experience right away without complex toolchain integration needs. I can simply focus on crafting excellent UI leveraging my React knowledge.
The reduced bundle sizes also provide a snappier user experience improving perceived performance – something vital for modern web experiences. While React offers ultimate customizability and flexibility, the out of the box efficiencies Next.js enable me to deliver complete applications significantly faster aligning well with my style.
So for me, Next.js effectively complements React expanding its capabilities for assembling full web applications. Of course developers and teams should assess their existing skills, needs and constraints before choosing. But I’ve found Next.js accelerates development tremendously vs React alone through its well-considered set of conventions and auto-optimizations.
Conclusion
Nuxt.js and Gatsby.js are both incredible modern web frameworks built around Vue and React respectively. They incorporate the latest advances delivering great performance, SEO effectiveness and amazing developer experiences.
Nuxt shines when building fast, universal Vue applications taking advantage of advanced server-side rendering and Vuex state management right out of the box. Its conventions accelerate development.
Gatsby wins where pre-rendered blazing-fast static websites are key leveraging its automatic performance optimizations, React’s capabilities and the rich data plugin ecosystem.
So your technology preferences, comfort with GraphQL vs traditional approaches and website goals should guide your choice between these two frameworks. Evaluate teams skills, use case needs and your preferences to determine the right fit.
Both continue strong momentum so you can be confident choosing either Nuxt or Gatsby for crafting amazing next-generation web experiences.